What is it and how it works?
The Call Stack is like a to-do list. Example in the code:
1
2
3
4
5
6
7
8
9
|
function firstFunction() {
return "First Function";
}
function secondFunction() {
return firstFunction() + " Second Function";
}
console.log(secondFunction());
|
This is our call stack:
1
2
3
4
5
6
|
# #
# #
# #
# #
# #
############
|
When we call secondFunction, it is added to stack:
1
2
3
4
5
6
|
# #
# #
# #
# #
# secondFunction() #
####################
|
Then, secondFunction call firstFunction, so, this is added to stack:
1
2
3
4
5
6
|
# #
# #
# #
# firstFunction() #
# secondFunction() #
####################
|
When the firstFunction is completed (in this case make the return) it leaves the stack:
1
2
3
4
5
6
|
# #
# #
# #
# #
# secondFunction() #
####################
|
But now, the secondFunction is completed too, so, it has to leave the stack:
1
2
3
4
5
6
|
# #
# #
# #
# #
# #
############
|
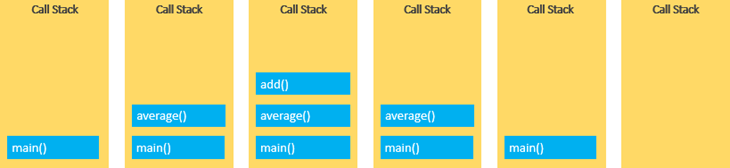
RangeError
If we do a function loop, we will have a RangeError: Maximum call stack size exceeded:
1
2
3
4
5
6
7
8
9
|
function firstFunction() {
secondFunction();
}
function secondFunction() {
firstFunction();
}
secondFunction();
|
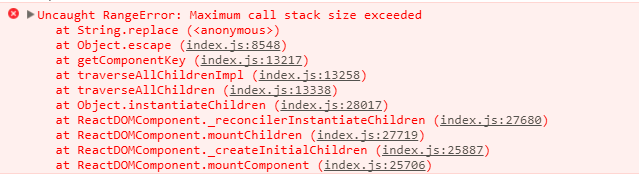